CodingHome
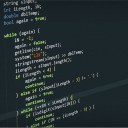
View pop-up format
Disclaimer
We do not take responsibility for any damage, or legal issues, done with these files here at AT Products LLC, Ethical Hacking Society, The Script Community, CodingHome, or Noodle Hackerspace.
Use a virtual machine if it's a computer virus, and never open them on your physical machine. As a pre-caution, download them on your VM.
Viruses & Malware
Meme_Coin (ZIP) Meme_Coin (EXE) My_App (ZIP) Myballzhert (ZIP) by Dream3456789
Password: "mysubsarethebest" (Myballzhert.zip)
USB & Internet Disabler (BAT) Unkill Tool for Disabler (BAT) by CodingLive
Trojan_Spyer (EXE) by MagSam2
Nuking Discord Bot (ZIP) Scam (ZIP) by the pfp is for nuking kpopserver
BFF_DoS (exe) Code (TXT) Baby (BAT) BabyCodes (TXT) by Corrupt Assassin
Code Samples
ISS Obfuscator (Python 3.9) by vesper
Snake Game in One Line of Code (Python) by ByThon
Encochat by CodingLive
Apps & Software
SMS Bomb (APK) by CodingLive
Python Basics
Comments
You can write text that the program will ignore by beginning the line with a #, this helps with reminding you what certain code does or for explaining purposes.
py
# This is a comment
Variables can store data of different types, and different types can do different things.
Python has the following data types built-in by default, in these categories:
Text Type: str
Numeric Types: int, float, complex
Sequence Types: list, tuple, range
Mapping Type: dict
Set Types: set, frozenset
Boolean Type: bool
Binary Types: bytes, bytearray, memoryview
We will go more in-depth on some of these types in the next few paragraphs.
You can print the data type of a variable with the type() function:
py
x = 5
print(type(x))
Strings
Strings are surrounded by either single quotation marks, or double quotation marks.
py
x = "hello"
y = 'hello'
# single quotations and double quotation marks are the same
x == y # returns True
You can assign a multiline string to a variable by using three quotes:
py
x = """The FitnessGram Pacer test is a multistage aerobic capacity test that progressively gets more difficult as it continues. The 20 meter Pacer test will begin in 30 seconds."""
You can check the length of a string using the len() function:
py
x = "hello"
print(len(text)) # returns 5
Numbers
There are 2 primary, int
and float
py
x = 2 # int
y = 2.8 # float
To verify the type of an object in Python, use the type() function:
py
print(type(x))
print(type(y))
Int, or integers, are whole numbers, positive or negative, without decimals.
Float is a number, positive or negative, containing one or more decimals.
Both strings and numbers are built-in data types.
C++ Basics
Strings
A string is a sequence of characters representing text. Strings are typically enclosed in quotation marks and can be stored in variables for later use in a program.
#include <ostream>
#include <string>
int main()
{
// Declare a string variable
std::string str = "codinghome";
}
Integers and Floating Point Numbers
Simply said, Integers are used for numbers without a decimal, and Float is used for numbers with decimals, simply said. There is more to that but we are keeping it simple.
#include <iostream>
int main()
{
// Declare an integer variable
int num = 10;
// Declare a floating-point variable
float fnum = 10.5;
}
Printing an output to the terminal/console or whatever you want to call it.
#include <iostream>
int main()
{
// Declare an integer variable
int num = 10;
// Declare a floating-point variable
float fnum = 10.5;
std::cout << "num: " << num << std::endl; // <-- Prints the integer Variable (Variable num)
std::cout << "fnum: " << fnum << std::endl; // <-- Prints the floating point Variable(Variable fnum)
}
HTML Basics
Example Document
<!DOCTYPE html>
<html>
<body>
<h1>My first Heading</h1>
<p>My first paragraph</p>
</body>
</html>
The <!DOCTYPE>
declaration represents the document type, and helps browsers to display web pages correctly. It must only appear once, at the top of the page (before any HTML tags). The <!DOCTYPE>
declaration is not case sensitive.
HTML Headings
HTML headings are defined with the <h1>
to <h6>
tags.
<h1>
defines the most important heading. <h6>
defines the least important heading:
<h1>Big</h1>
<h6>Small</h6>
HTML Paragraphs
HTML paragraphs are defined with the <p> tag:
<p>This is a paragraph.</p>
<p>This is another paragraph.</p>
HTML Links
HTML links are defined with the <a> tag:
<a href="https://example.com">This is a link</a>
HTML Images
HTML images are defined with the <img> tag. The source file (src), alternative text (alt), width, and height are provided as attributes:
<img src="example.jpg" alt="example.com" width="104" height="142">
CSS Basics
Example Document
h1 {
color: black;
text-align: center;
font-size: 26px;
font-family: verdana;
}
<p style="font-size:10px">
To put CSS files in HTML, use... <link rel="stylesheet" href="style.css">
CSS Rulesets
Selector - p {
Property - color: red;
Declaration - }
Properties - With these, you can style a HTML element, like for example; color.
Property Value - This chooses one of the possible options for a given property, like red for color.
You can also write CSS like html{color:red;}
You can also put styles in HTML elements. <h1 style="font-color:white;">
You can also select multiple elements at once like...
h1, h4, h6, h2 {
color: red;
}
Text Elements
font-size: 10px;
- Text size, can be in pixels.
font-family: verdana;
- Fonts
text-align: center;
- Can align to the left side, right side, or in the center of the webpage.
text-shadow: 3px black
- Shadow of text. Can be in pixels and colors.
Padding Elements
padding-top: 50px;
- Separation. Can be top, left, right, and the bottom in pixels.
margin: 50px;
- A margin. Can be in pixels.
border-width: 5px;
- Border width. Can be thin, medium, thick, or just pixels.
border-style: dotted;
- Border style. Can be dotted, dashed, solid, double, groove, ridge, inset, outset, none, or hidden. Can also be colored.
width=500px;
- Width of the element. Can be pixels or percentages.
Color Elements
color: red;
- Color of the element, can be hexadecimal, or RGB.
background-color: white;
- Background color of the element.
Extra: Centering Images
img {
margin: 0 auto;
display: block;
}
JavaScript Basics
Including JavaScript in an HTML Page
<script type="text/javascript">
//JS code goes here
</script>
Call an External JavaScript File
<script src="myscript.js"></script>
Including Comments
Single line comments - // Comment
Multi-line comments - /* comment here */
Variables
var, const, let
var
— The most common variable. Can be reassigned but only accessed within a function. Variables defined with var move to the top when code is executed.
const
— Can not be reassigned and not accessible before they appear within the code.
let
— Similar to const, however, let variable can be reassigned but not re-declared.
Data Types
Numbers —var age = 23
Variables —
var x
Text (strings) —
var a = "init"
Operations —
var b = 1 + 2 + 3
True or fase statements —
var c = true
Constant numbers —
const PI = 3.14
Objects —
var name = {firstName:"John", lastName:”Doe"}
Objects Example
var person = {
firstName:"John",
lastName:"Doe",
age:20,
nationality:"American"
};